Normally actions are integrated into workflows and invoked from there. The way to invoke a workflow from an action is rather unusual and less common. However, this approach offers the advantage of complete embedding in a programming language environment. All the possibilities offered by coding can therefore be used. E.g. for unit tests, this means that quality assurance measures can also be implemented for workflows on this way.
This post shows examplary how a workflow can be invoked up from an action. For this purpose we take the workflow 'Get virtual machines by name', which returns a list of virtual machines from all registered vCenter server hosts that match the provided expression. This workflow has the following Inputs/Outputs interface:
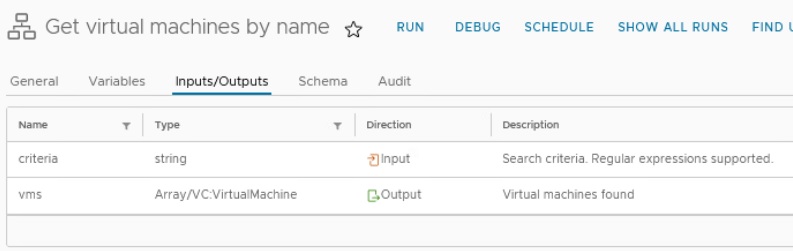
That's all we need to know to execute this workflow. The following action shows how to ...
- define the workflow,
- get the workflow,
- define the inputs,
- execute the workflow,
- wait until the workflow is terminated and
- get the outputs.
A simple sequence that can be used again and again, with the exception of the Inputs and Outputs, which must be adapted to the corresponding workflow.
It is important that the Inputs and Outputs must be defined with their exact names that they have in the interface definition.
The following image sequence shows the result of executing the 'Get virtual machines by name' workflow with the criteria 'ubuntu' in the HOL.
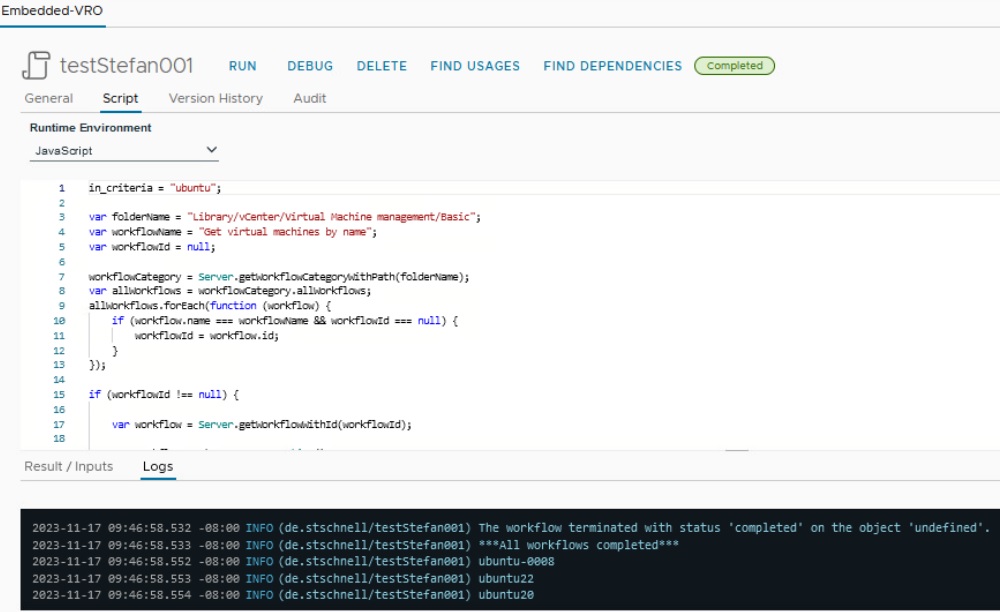

Conclusion
As we can see, executing workflows from actions is very simple. This opens up many opportunities for us, e.g. as already mentioned, the integration of workflows in unit tests. The detailed logging of the workflows helps us to analyze the results quickly and clearly, to detect errors in this context.