VMware Aria Automation offers a polyglot programming environment, with JavaScript, PowerShell and Python as programming languages. We have the opportunity to expand this range to include additional programming languages. This post shows how the
C# programming language can be seamlessly used. In addition an approach is shown, to save the C# code in Aria Automation and to executes it directly. To realize this we use the current PowerShell 7.3 container, of release 8.13.1, that contains the
Common Language Runtime (CLR) 7.0.9 dotNET run-time environment.
Here are the necessary actions required for this:
- testCSharp: Contains the C# source code.
- getActionAsText: JavaScript that reads the C# source code.
- executeCSharp: PowerShell source to execute the C# source code.
- invokeCSharp: JavaScript that invokes the C# source code.
To get the source code move your mouse pointer into the source code section and press on the upper right side the copy button. This copies the source code to the clipboard and now it can be pasted and saved e.g. in an editor on the target system. Or download it from my
GitHub account. Here the
corresponding post in the VMware community.
Save C# Code as Action
In the first step we build an action, who we called testCSharp, in which we store the C# code to be executed. We simply save the C# source code here.
using System;
using System.Management.Automation;
public class EntryPoint {
public static object main(dynamic arguments) {
// Console.WriteLine("Hello World!");
return "Hello World";
}
}
|
As we can see, this code doesn't do anything special, it just returns Hello World. Not imaginative, but sufficient for our example. The class and method names are also important here, because these are required for the invoke later.
Read Action as Text
In the second step we build a JavaScript action, called getActionAsText. As the name suggests, the content of an action is read out as text. With this action we read the C# code and pass it for execution.
Here all actions are filtered, first by the module name and then by the action name, to return the content of the specified action.
PowerShell to Execute C#
In the third step we build a PowerShell action to execute the C# code.
<#
# @param {string} csharpSource
# @param {Properties} arguments
# @returns {Properties}
#
# Memory limit (MB) 512
# Timeout (seconds) 360
#
# @author Stefan Schnell <mail@stefan-schnell.de>
# @license MIT
# @version 0.2.0
#
# Checked with Aria Automation 8.12.0 and 8.14.0
#>
function Handler($context, $inputs) {
Add-Type -TypeDefinition $inputs.csharpSource -Language CSharp;
$result = [EntryPoint]::main($inputs.arguments);
$output = @{
status = "done"
result = $result
};
return $output;
}
|
This executes the C# code. The class and method names appear again here. They are added with the
Add-Type cmdlet and then called.
Invoke C# Code
In the fourth and final step we build an action to invoke the C# code.
/**
* Invokes C# code.
*
* @param {string} in_moduleName - Module name which contains the action
* @param {string} in_actionName - Action name which contains the C# code
* @param {Properties} in_arguments - Arguments to be passed to the C# code
* @param {boolean} in_showOutput - Flag whether output should be displayed
* @returns {string} result - Result of C# code execution
*
* @author Stefan Schnell <mail@stefan-schnell.de>
* @license MIT
* @version 0.2.0
*
* @example
* var in_moduleName = "de.stschnell";
* var in_actionName = "testCSharp";
* var in_showOutput = false;
*
* Checked with Aria Automation 8.12.0 and 8.14.0
*/
var _invokeCSharpNS = {
main : function(moduleName, actionName, arguments, showOutput) {
try {
var csharpSource = System.getModule("de.stschnell")
.getActionAsText(moduleName, actionName);
if (showOutput) {
System.log(csharpSource);
}
var csharpExecute = System.getModule("de.stschnell")
.executeCSharp(csharpSource, arguments);
if (showOutput) {
System.log(String(csharpExecute.result));
}
return String(csharpExecute.result);
} catch (exception) {
System.log(exception);
}
}
}
if (
String(in_moduleName).trim() !== "" &&
String(in_actionName).trim() !== ""
) {
return _invokeCSharpNS.main(
in_moduleName,
in_actionName,
in_arguments,
in_showOutput
);
} else {
throw new Error("in_moduleName or in_actionName argument can not be null");
}
|
This is the "bracket" which invokes all the other modules. It calls the action getActionAsText, it executes the C# code and then it delivers the result.
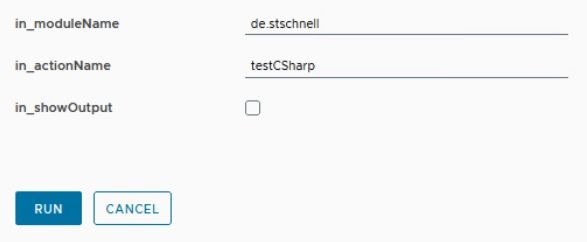
Conlusion
This approach shows that it is very easy to integrate C# directly into VMware Aria Automation, and also save the source code. A little combination of the possibilities of the polyglot programming environment allows us to do this.
This site is part of
blog.stschnell.de