Java allows to execute multiple threads concurrently. We can use this approach to enable parallel execution of functions in the JavaScript runtime environment also. This example shows how parallel execution can be easily implemented in JavaScript. In this context it is important to note that parallel threads cannot write anything directly to the standard output stream of VMware Aria Automation, because it cannot be displayed by using the method System.log, if an output is necessary.
Hint: For this approach it is necessary to set the system property com.vmware.scripting.javascript.allow-native-object to true or add in the shutter file the java.* and org.* package, or the relevant subsets of it.
To get the source code move your mouse pointer into the source code section and press on the upper right side the copy button. This copies the source code to the clipboard and now it can be pasted into an action and saved on the target Aria Automation system.
Thread Class with Output Redirecting
First a look at an implementation using the Java Thread class. In this case the
standard output stream is to be redirected, as long as a thread is running. In this example this was implemented for reasons of clarity. Once this has happened, the thread can be started asynchronously. In this case the asynchronous function counts every second, for ten seconds. The caller waits four seconds and then it interrupts the thread by using a global control variable. Finally the content of the file, which contains the redirected output stream, are read and displayed.
The following image shows the result of an execution in Aria Automation.
Executor Interface with Logger
The following source code shows another approach, without the redirecting of the standard output stream. VMware Aria Automation uses the
Simple Logging Facade for Java (SLF4J). Here a connection to the existing log is established. The
Java Executor interface is also used. It is a simple standardized interface for defining custom thread-like subsystems, including thread pools, asynchronous I/O and lightweight task frameworks.
The following image shows the result of an execution in Aria Automation.
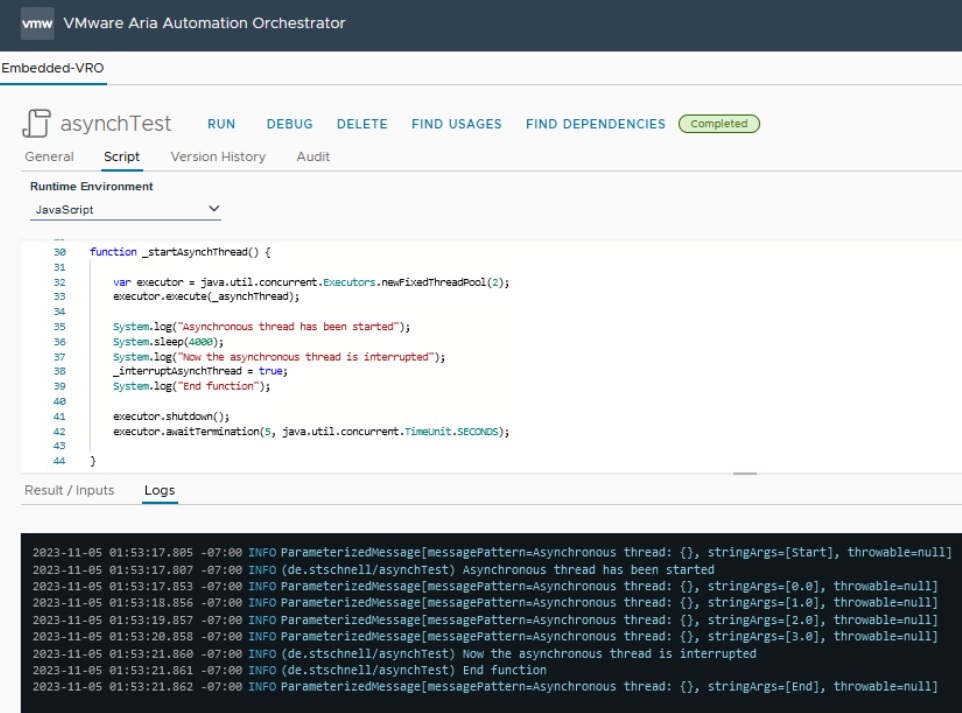
As can be seen here, the direct SLF logging takes a specific format, the parameterized log message.
Executor Interface with Logger and Parameter Passing
The following source extends the previous. Five threads are started in parallel and an identification number is passed as a parameter to each thread.