Test Driven Development (TDD) is a software development approach. Here the test is coded first before the actual code is programmed. TDD uses the repetition of short development cycles, so that building and testing are combined. A good test coverage helps to check code changes fast to ensure that the required functionality is still guaranteed. This methodology improves the quality of the software. To catch a bug in your development system is much better than by your customer in production. This blog post describes how the TDD approach can be used in Orchestrator.
Test Driven Development (TDD) with the Orchestrator
The TDD approach presented here bases on the
Aria Automation Assert library, which was built also for this purpose.
Below are the process steps of a unit test in the Orchestrator of VMware Aria Automation:
- Build action to be tested
This step adds an action.
- Define the interface of the action to be tested
Here the input and output parameters are added.
- Build unit test
This step adds an unit test action.
- Code unit test
The unit test is programmed here. After that the first test can be done at this point, in which all tests fail naturally.
- Code the action to be tested
This is where the actual functionality is programmed.
- Execute unit test
In this step the unit test is executed and the result is displayed. If the expected result is not displayed, an iterative process begins.
For the following example we use very trivial code, because the focus of this post is the TDD approach.
Build Action to be Tested
At the first step we create only an action, with a name, description and version number. The optional description and versioning should always be set, this helps others and often yourself at a later point in time.
Define the Interface of the Action to be Tested
At the second step we create the input parameters, with a name, data type and description, and define the return data type. The optional parameter description should also be set here.
This test example simply add two numbers and return the result.
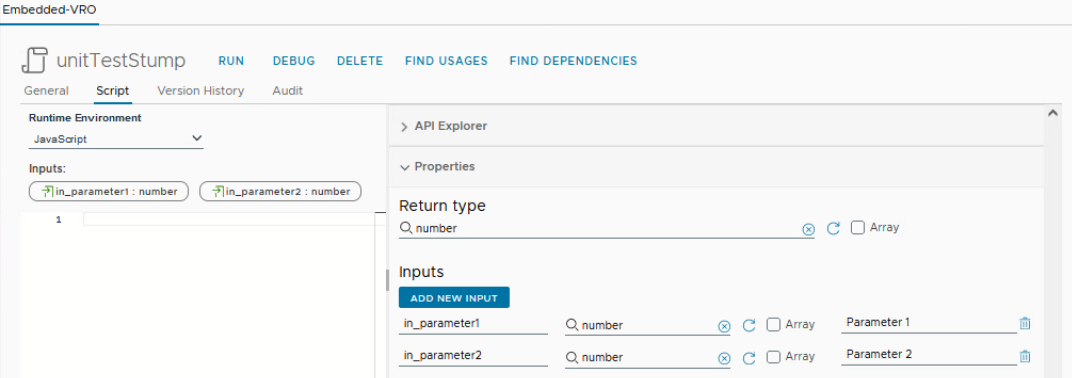
If this step has been completed, we have an executable action. This does not deliver a result, but it can be executed. This is the basis for executing a unit test with this action.
Build Unit Test
At the third step we create an action which is the unit test. It is not necessary to define an interface, because all data to be processed are included in the unit test code.
Code Unit Test
At the fourth step the unit test is programmed. Here we check the data type of the return value and the sum of an addition of two numbers.
Our assertion is that the sum of 20 plus 22 is 42, of data type number.
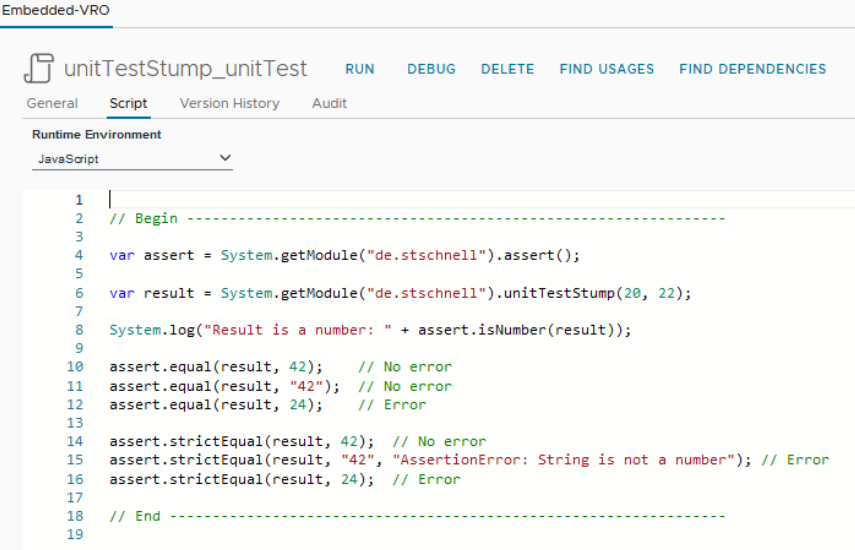
If we now run this unit test, it would return an error for each assertion.
Code the Action to be Tested
At the fifth step the action to be tested is programmed.
This test example simply add two numbers and return the result.
Execute Unit Test
At the final and sixth step we can now execute the unit test.
As we can see, our assertion that 20 plus 22 is 42 is confirmed, also the fact that 42 is a number.
Conclusion
We see that the TDD approach can also be technically used in VMware Aria Automation without any problems. The procedure does not differ really in any way from the usual. It was simply adapted a little bit to the specific conditions. TDD is a mindset that needs to be practiced. This is probably where the higher barriers will lie, in changing the coding approach, in rethinking to start with the test first and define the expected results. Routine must be established with this methodology.