C and C++ have been very popular programming languages for a long time and still are today. Many consolidated program approaches and libraries exists. With
Emscripten is it possbile to transfer existing C / C++ projects into
WebAssembly. This kind of output, which is generated by Emscripten, can be used seamlessly with
Node. And Node is one of the platforms that Aria Automation offers as runtime engine. This is definitely worth a look to see if C / C++ programs can be made to work with Aria Automation.
Use C / C++ Language via Node
Preparations
The download, installation and setup are described in detail in the
section of the same name on Emscripten's site. It is very simple and works without any problems. After veryfying the installation, Emscripten can be used.
C Code
To check it I use this tiny C program with two functions. The first function hello outputs Hello World and the second function int_sqrt calculates the squareroot of the given number. The second function is part of the Emscripten example,
how to interact with code.
#include <stdio.h>
#include <string.h>
#include <math.h>
#include <emscripten.h>
EMSCRIPTEN_KEEPALIVE
void hello(char *name) {
if (name == NULL) {
printf("Hello World from C Language!\n");
} else {
printf("Hello %s from C Language!\n", name);
}
}
EMSCRIPTEN_KEEPALIVE
int int_sqrt(int x) {
return sqrt(x);
}
|
Save this file as helloWorld.c.
Compile the C Code to WebAssembly
Now we compile the code with the following command:
emcc helloWorld.c -o helloWorld.js -s EXPORTED_FUNCTIONS=_hello,_int_sqrt -s EXPORTED_RUNTIME_METHODS=ccall,cwrap
After compilation we have two new files, helloWorld.js and helloWorld.wasm.
Aria Automation Handler
In the next step we build a handler program to call the functions on the different ways, direct call, ccall and cwrap. A detailed explanation can also be found in the
Interacting with Code section. However, the calls and returns are very simple to understand.
/**
* Node.js test action to check Emscripten compiler to WebAssembly
*
* Checked with VMware vRealize Automation 8.5.1 with Node.js 12.22.1
* and VMware Aria Automation 8.18.0 with Node.js 20.11.0
*
* @param sqrtFrom {number}
* @returns CompositeType(
* status {string},
* result {number}
* ):test
*/
exports.handler = (context, inputs, callback) => {
let sqrtFrom = inputs.sqrtFrom;
const helloWorld = require("./helloWorld.js");
let result = helloWorld.onRuntimeInitialized = () => {
// Try C function hello
helloWorld._hello();
helloWorld.ccall("hello", null, ["string"], ["Gabi"]);
const hello = helloWorld.cwrap("hello", null, ["string"]);
hello("Stefan");
// Try C function int_sqrt
let result = helloWorld._int_sqrt(4);
console.log(result);
console.log(helloWorld.ccall("int_sqrt", "number", ["number"], [25]));
const intSqrt = helloWorld.cwrap("int_sqrt", "number", ["number"]);
console.log(intSqrt(36));
let fromSqrt = intSqrt(sqrtFrom);
console.log(fromSqrt);
callback(undefined, {status: "done", result: fromSqrt});
}
}
|
Zip
Now we build a zip file as it is described in the VMware documentation. All files can be packed together, directories are not necessary.
Execution
Now we can add the zip file in the Orchestrator Node.js runtime and set the inputs and return type.
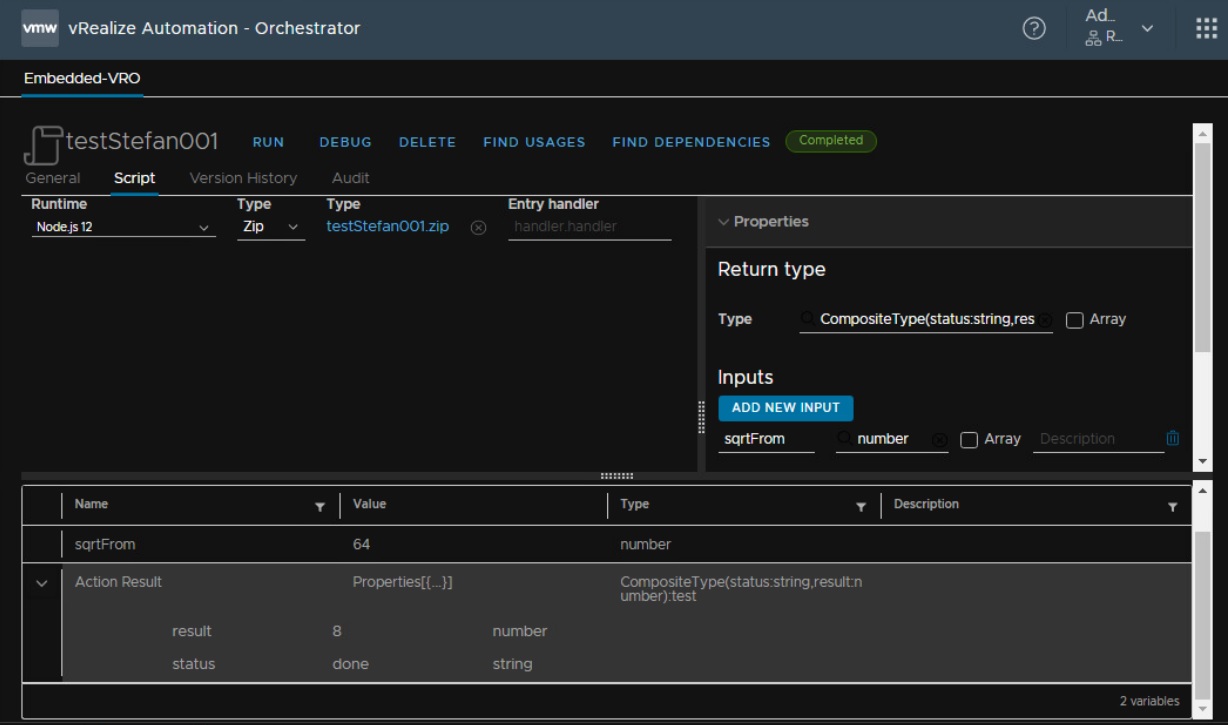
Everything works well, as we can see.
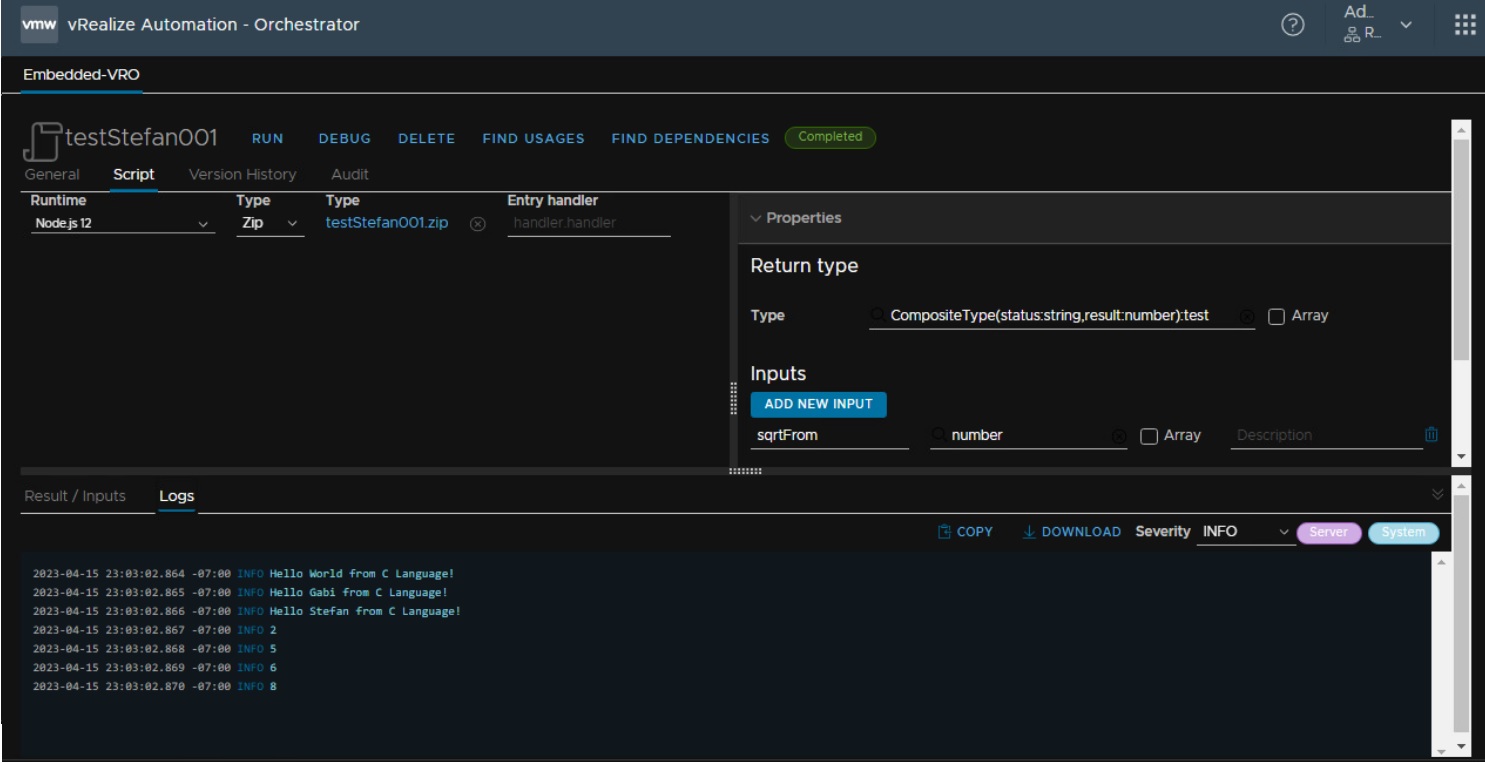
Three times hello call delivers the expected results and four times int_sqrt delivers the expected results. Input and output values are also processed and returned correctly.
Conclusion
With Emscripten it is very easy to convert C / C++ functions into WebAssembly and with Node it is also very easy to use WebAssembly inside Aria Automation. This approach offers us the possibility to use C / C++ code seamlessly in Aria Automation. It opens up interesting development perspectives, even if there are
limitations. One of the most famous projects that have been implemented with Emscripten to JavaScript is
SQLite. This clearly demonstrates the power of this approach.