Lua is a cross-platform scripting language designed as an extension language to embed it in other applications. It is part of the VMware Aria Automation installation. When the command lua is entered in a console, the Lua shell opens.
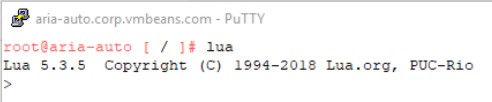
This makes it possible to integrate and use Lua in the context of programming Aria Automation. The Command class can be used to call it.
Integrate Lua Scripting Language
The following example builds the helloWorld.lua script in the temporary directory (lines 10 to 15). The script simply prints a greeting and the Lua version. Then this script is executed using the command class (lines 17 and 18) and the result is displayed (line 19).
/**
* @author Stefan Schnell <mail@stefan-schnell.de>
* @license MIT
* @version 0.1.0
*
* Set in the VMware Control Center the system property
* com.vmware.js.allow-local-process to true.
*/
var luaFileName = System.getTempDirectory() + "/helloWorld.lua";
var luaFile = new FileWriter(luaFileName);
luaFile.open();
luaFile.clean();
luaFile.write("print(\"Hello World from \" .. _VERSION);");
luaFile.close();
var commandLua = new Command("lua " + luaFileName);
commandLua.execute(true);
System.log(commandLua.output);
|
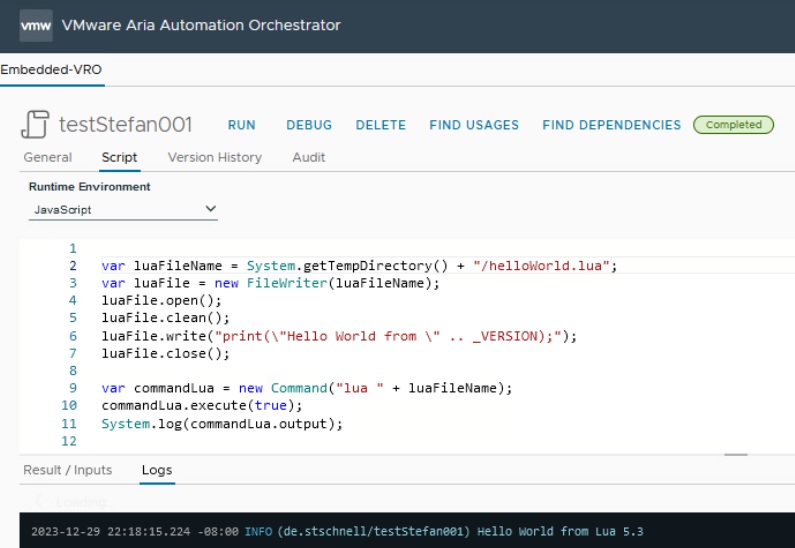
In the next example we start Lua inside Lua. With the command io.popen the program lua starts in a separated process and returns the Lua version, which is read by file:read and output using print. The sequence is equivalent to the previous example, the file getVersion.lua is written (lines 10 to 15), executed (lines 17 and 18) and the result is displayed (line 19).
/**
* @author Stefan Schnell <mail@stefan-schnell.de>
* @license MIT
* @version 0.1.0
*
* Set in the VMware Control Center the system property
* com.vmware.js.allow-local-process to true.
*/
var luaFileName = System.getTempDirectory() + "/getVersion.lua";
var luaFile = new FileWriter(luaFileName);
luaFile.open();
luaFile.clean();
luaFile.write("file = io.popen(\"lua -v\");print(file:read());");
luaFile.close();
var commandLua = new Command("lua " + luaFileName);
commandLua.execute(true);
System.log(commandLua.output);
|
Using Some Extensions
Below we will use some extensions that make the use much easier, of Lua in particular and code from other programming languages in general. With this scheme we can integrate any programming language. Here are the necessary actions required for this:
Advanced Operating System Command Execution
Here is another example in which the greeting and the version are output. However, the operating system command is executed here using via an extended implementation of operating system command call, with more options. This approach also works without any problems.
/**
* @author Stefan Schnell <mail@stefan-schnell.de>
* @license MIT
* @version 0.1.0
*
* Set in the VMware Control Center the system property
* com.vmware.scripting.javascript.allow-native-object to true.
*/
try {
var luaFileName = System.getTempDirectory() + "/helloWorld.lua";
var luaFile = new FileWriter(luaFileName);
luaFile.open();
luaFile.clean();
luaFile.write("print(\"Hello World from \" .. _VERSION);");
luaFile.close();
// var command = [ "lua", "-v" ];
var command = [ "lua", luaFileName ];
var output = executeCommand(command, 5000).output;
System.log(output);
} catch (exception) {
System.error(exception);
}
|
Read Action as Text and Store it as File
It is also possible to save the source code as an action, read the content of this action as a text and save it as a file. I use this approach e.g. in the context of the
TypeScript integration scenario or the
C# integration scenario.
After these actions have been created, the following Lua source code can be saved as an action.
print("Hello World from " .. _VERSION);
|
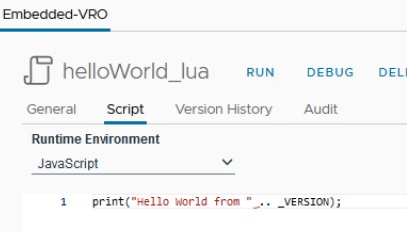
Now the Lua source code can be executed.
/**
* @author Stefan Schnell <mail@stefan-schnell.de>
* @license MIT
* @version 0.1.0
*/
System.getModule("de.stschnell").writeActionAsFileInTempDirectory(
"de.stschnell",
"helloWorld_lua",
"helloWorld.lua"
);
var luaFileName = System.getTempDirectory() + "/helloWorld.lua";
var output = System.getModule("de.stschnell").executeCommand(
["lua", luaFileName], 5000
).output;
System.log(output);
|
Conclusion
This is an additional integration scenario that shows us how easy it is to integrate Lua scripting language. It is available as standard and can be used without any problems seamlessly with the JavaScript runtime environment in VMware Aria Automation.
This site is part of
blog.stschnell.de